Setting up a new React Native app can be overwhelming, especially when integrating modern tools like TailwindCSS for styling. This guide will walk you through the step-by-step process of creating an Expo project and configuring NativeWind, a TailwindCSS implementation for React Native. Whether you're a seasoned developer or just getting started, this tutorial has you covered.
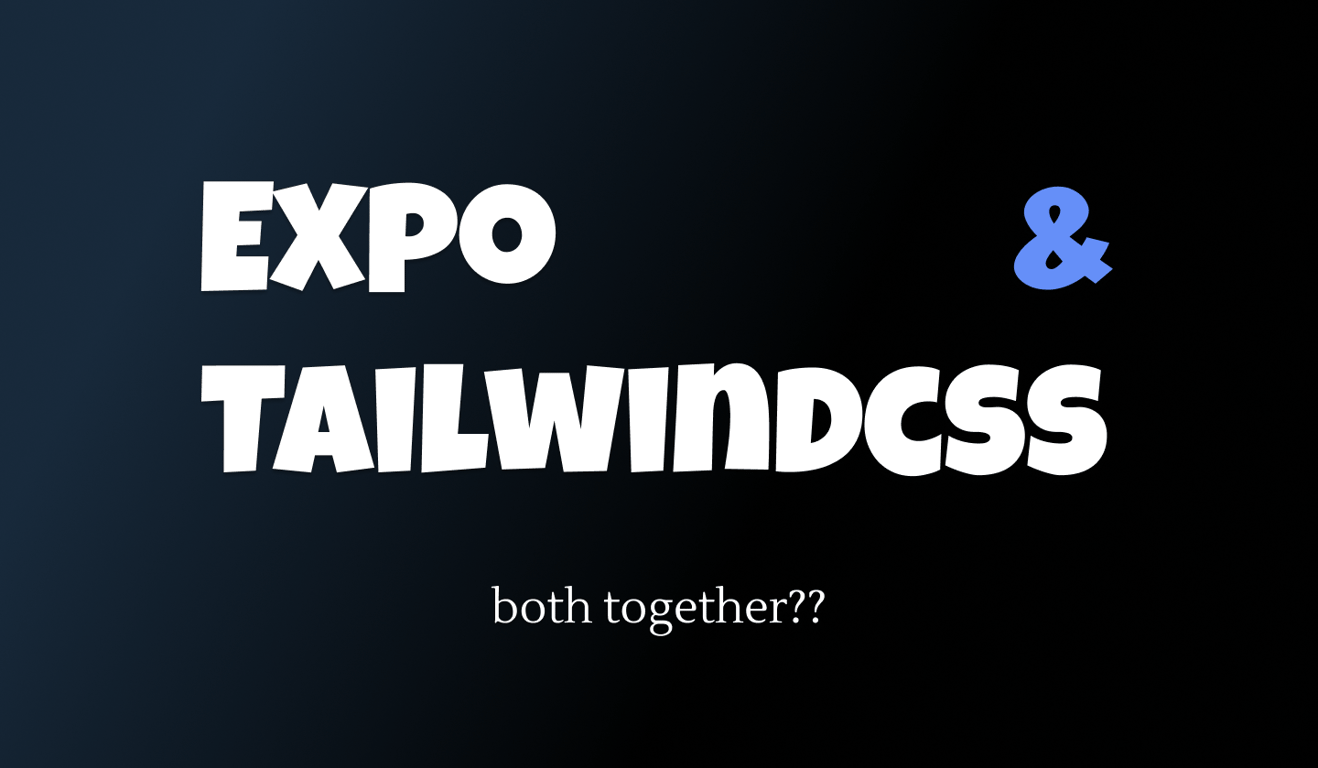
Why Choose Expo and NativeWind?
Benefits of Expo
Expo simplifies React Native development by providing:
Cross-platform support: Build for Android, iOS, and web with a single codebase.
Built-in tools: Includes Expo CLI for seamless app management.
TypeScript configuration: Pre-configured support for TypeScript.
Why NativeWind?
NativeWind brings the power of TailwindCSS to React Native, offering:
Utility-first styling: Simplify your styles with reusable utility classes.
Consistency: Unified styling for all platforms.
Ease of use: No need for complex configurations.
Let’s dive in!
Step 1: Create a New Expo Project
Start by creating a new React Native project with Expo:
npx create-expo-app@latest .
What This Command Does:
Initializes a new project with the
expo
package.Includes a tab navigator from Expo Router for basic navigation.
Configures TypeScript by default.
For more details, check out the official Expo tutorial.
Optional: Reset the Project
If you need a fresh start, reset your project using:
npm run reset-project
Start the App
To run your app and view it in action:
npx expo start
If everything works fine, you’re ready to integrate NativeWind.
Step 2: Install NativeWind (TailwindCSS for React Native)
Install Required Dependencies
Run the following command to install NativeWind and its dependencies:
npm install nativewind tailwindcss react-native-reanimated react-native-safe-area-context
Generate Tailwind Config File
Create the Tailwind configuration file:
npx tailwindcss init
Configure tailwind.config.js
Update the file to include the paths to your components and enable NativeWind:
/** @type {import('tailwindcss').Config} */
module.exports = {
content: ["./app/**/*.{js,jsx,ts,tsx}"],
presets: [require("nativewind/preset")],
theme: {
extend: {},
},
plugins: [],
};
If you’re using a src
folder, replace ./app/
with ./src/
in the content
field.
Create Global Styles
Add a global.css
file with the following content:
@tailwind base;
@tailwind components;
@tailwind utilities;
Step 3: Configure Babel and Metro
Babel Configuration
Create a babel.config.js
file to support NativeWind:
module.exports = function (api) {
api.cache(true);
return {
presets: [
["babel-preset-expo", { jsxImportSource: "nativewind" }],
"nativewind/babel",
],
};
};
Customize Metro Configuration
Run the following command to create a metro.config.js
file:
npx expo customize metro.config.js
Update the file to integrate NativeWind:
const { getDefaultConfig } = require('expo/metro-config');
const { withNativeWind } = require("nativewind/metro");
/** @type {import('expo/metro-config').MetroConfig} */
const config = getDefaultConfig(__dirname);
module.exports = withNativeWind(config, { input: "./global.css" });
Step 4: Final Setup in App Files
Import Global CSS in Layout
In your app/_layout.tsx
file, add this import at the top:
import "../global.css";
Test NativeWind
To confirm everything works, update your index.tsx
file with a sample component:
<Text className="bg-orange-500 p-4 font-bold mt-2 rounded-lg">
NativeWind is Working
</Text>
Run the App
Finally, run the app to see the magic:
npx expo start
Troubleshooting Tips
Metro Bundler Issues:
Ensure the
metro.config.js
file is correctly configured.
NativeWind Classes Not Working:
Verify the
content
field intailwind.config.js
matches your project structure.
Reanimated Errors:
Install and configure
react-native-reanimated
as per Expo’s documentation.
Conclusion
Congratulations! Your React Native app is now set up with Expo and NativeWind, leveraging the power of TailwindCSS for styling. With this configuration, you can build stunning, responsive designs quickly and efficiently.
Happy coding! 🚀